In this post, I will discuss about hosting node.js application in IIS on Windows using iisnode project. OK, let’s begin.
First thing you need to do is install iisnode project side to get the module and samples installed on your Windows box with IIS7 enabled. The hello world sample consists of two files: hello.js and web.config.
This is the hello.js file from the helloworld sample:
var http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, world! [helloworld sample]');
}).listen(process.env.PORT);
You will notice that the only difference between this code and the hello world sample from the front page of http://nodejs.org is in the specification of the listening address for the HTTP server. Since IIS controls the base address for all HTTP listeners, a node.js application must use the listen address provided by the iisnode module through the process.env.PORT environment variable rather than specify its own.
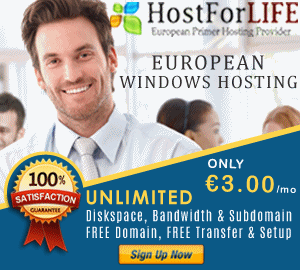
The web.config file is required to instruct IIS that the hello.js file contains a node.js application. Otherwise IIS would consider this file to be client side JavaScript and serve it as static content. The web.config designates hello.js as a node.js application by scoping the registration of the handler in the iisnode module to that file only:
<configuration>
<system.webServer>
<handlers>
<add name="iisnode" path="hello.js" verb="*" modules="iisnode" />
</handlers>
</system.webServer>
</configuration>
This handler registration allows the same web site to contain other *.js files (e.g. jQuery libraries) that IIS will continue serving as static files.
The Advantages Using iisnode
WebSocket Support
As of version 0.2.x, iisnode supports hosting Websocket applications in IIS 8 on Windows Server 2012 and Windows 8. You can use any standard node.js modules that implement the Websocket protocol, including socket.io.
Scalability on multi-core servers
For every node.js application (e.g. hello.js above), iisnode module can create many node.exe process and load balance traffic between them. The nodeProcessCountPerApplication setting controls the number of node.exe processes that will be created for each node.js application. Each node.exe process can accommodate a configurable number of concurrent requests (maxConcurrentRequestsPerProcess setting). When the overall concurrent active request quota has been reached for an application (maxConcurrentRequestsPerProcess * nodeProcessCountPerApplication ), the iisnode module starts rejecting new HTTP requests with a 503 (Server Too Busy) status code. Requests are dispatched across multiple node.exe processes serving a node.js application with a round-robin load balancing algorithm.
Auto-update
Whenever the JavaScript file with a node.js application changes (as a result of a new deployment), the iismodule will gracefully upgrade to the new version. All node.exe processes running the previous version of the application that are still processing requests are allowed to gracefully finish processing in a configurable time frame (gracefulShutdownTimeout setting). All new requests that arrive after the JavaScript file has been updated are dispatched to a new node.exe process that runs the new version of the application. The watchedFiles setting specifies the list of files iisnode will be watching for changes.
Changes in the JavaScript file are detected regardless if the file resides on a local file system or a UNC share, but the underlying mechanisms are different. In case of a local file system, an OS level directory watching mechanism is used which provides low latency, asynchronous notifications about file changes. In case of files residing on a UNC share, file timestamps are periodically polled for changes with a configurable interval (uncFileChangesPollingInterval setting).
Integrated debugging
With iisnode integrated debugging you can remotely debug node.js application using any WebKit-enabled browser.
Access to logs over HTTP
To help in ‘console.log’ debugging, the iisnode module redirects output generated by node.exe processes to stdout or stderr to a text file. IIS will then serve these files as static textual content over HTTP. Capturing stdout and stderr in files is controlled with a configuration setting (loggingEnabled). If enabled, iisnode module will create a per-application special directory to store the log files. The directory is located next to the *.js file itself and its name is is specifed with the logDirectoryName setting (by default “iisnode”). The directory will then contain several text files with log information as well as an index.html file with a simple list of all log files in that directory. Given that, the logs can be accessed from the browser using HTTP: given a node.js application available at http://mysite.com/foo.js, the log files of the application would by default be located at http://mysite.com/iisnode/index.html.
The logDirectoryName is configurable to allow for obfuscation of the log location in cases when the service is publicly available. In fact, it can be set to a cryptographically secure or otherwise hard to guess string (e.g. GUID) to provide a pragmatic level of logs privacy. For example, by setting logDirectoryName to ‘A526A1F2-4E22-4488-B930-6A71CC7649CD’ logs would be exposed at http://mysite.com/A526A1F2-4E22-4488-B930-6A71CC7649CD/index.html.
Log files are not allowed to grow unbounded. The maxLogFileSizeInKB setting controls the maximum size of an individual log file. When the log grows beyond that limit, iisnode module will stop writing to that file and create a new log file to write to. To avoid unbounded growth of the total number of log files in the logging directory, iisnode enforces two additional quotas. The maxLogFiles setting controls the maximum number of log files that are kept. The maxTotalLogFileSizeInKB controls the maximum total size of all logs files in the logging directory. Whenever any of the quotas are exceeded, iisnode will remove any log files not actively written to in the ascending order of the last write time.
Note that this design of the logging feature allows the node.js application to be scaled out to multiple servers as long as the logging directory resides on a shared network drive.
Side by side with other content types
One of the more interesting benefits of hosting node.js applications in IIS using the iisnode module is support for a variety of content types within a single web site. Next to a node.js application one can host static HTLM files, client side JavaScript scripts, PHP scripts, ASP.NET applications, WCF services, and other types of content IIS supports. Just like the iisnode module handles node.js applications in a particular site, other content types will be handled by the registered IIS handlers.
Indicating which files within a web site are node.js applications and should be handled by the iisnode module is done by registring the iinode handler for those files in web.config. In the simplest form, one can register the iisnode module for a single *.js file in a web site using the ‘path’ attribute of the ‘add’ element of the handler collection:
<configuration>
<system.webServer>
<handlers>
<add name="iisnode" path="hello.js" verb="*" modules="iisnode" />
</handlers>
</system.webServer>
</configuration>
Alternatively, one can decide that all files in a particular directory are supposed to be treated as node.js applications. A web.config using the <location> element can be used to achieve such configuration:
<configuration>
<location path="nodejsapps">
<system.webServer>
<handlers>
<add name="iisnode" path="*.js" verb="*" modules="iisnode" />
</handlers>
</system.webServer>
</location>
</configuration>
One other approach one can employ is to differentiate node.js applications from client side JavaScript scripts by assigning a file name extension to node.js applications other than *.js, e.g. *.njs. This allows a global iisnode handler registration that may apply across all sites on a given machine, since the *.njs extension is unique:
<configuration>
<system.webServer>
<handlers>
<add name="iisnode" path="*.njs" verb="*" modules="iisnode" />
</handlers>
</system.webServer>
</configuration>
Output caching
IIS output caching mechanism allows you to greatly improve the throughput of a node.js application hosted in iisnode if the content you serve can be cached for a time period even as short as 1 second. When IIS output caching is enabled, IIS will capture the HTTP response generated by the node.js application and use it to respond to similar HTTP requests that arrive within a preconfigured time window. This mechanism is extremely efficient, especially if kernel level output caching is enabled.
URL Rewriting
The iisnode module composes very well with the URL Rewriting module for IIS. URL rewriting allows you to normalize the URL space of the application and decide which IIS handlers are responsible for which parts of the URL space. For example, you can use URL rewriting to serve static content using the IIS’es native static content handler (which is a more efficient way of doing it that serving static content from node.js), while only letting iisnode handle the dynamic content.
You will want to use URL rewriting for majority of node.js web site applications deployed to iisnode, in particular those using the express framework or other MVC frameworks.
Minimal changes to existing HTTP node.js application code
It has been the aspiration for iisnode to not require extensive changes to existing, self-hosted node.js HTTP applications. To that end, most applications will only require a change in the specification of the listen address for the HTTP server, since that address is assigned by the IIS as opposed to left for the application to choose. The iisnode module will pass the listen address to the node.exe worker process in the PORT environment variable, and the application can read it from process.env.PORT:
var http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('I am listening on ' + process.env.PORT);
}).listen(process.env.PORT);
If you access the endpoint created by the application above, you will notice the application is actually listening on a named pipe address. This is because the iisnode module uses HTTP over named pipes as a communication mechanism between the module and the worker node.exe process. One implication of this is HTTPS applications will need to be refactored to use HTTP instead of HTTPS. For those applications, HTTPS can be configured and managed at the IIS level itself.
Configuration with YAML or web.config
The iisnode module allows many of the configuration options to be adjusted using the iisnode.yml file or the system.webServer/iisnode section of web.config. Settings in the iisnode.yml file, if present, take precedence over settings in the web.config. Below is the list of options (most of which were described above) with their default values.
# The optional iisnode.yml file provides overrides of
# the iisnode configuration settings specified in web.config.
node_env: production
nodeProcessCommandLine: "c:\program files\nodejs\node.exe"
nodeProcessCountPerApplication: 1
maxConcurrentRequestsPerProcess: 1024
maxNamedPipeConnectionRetry: 100
namedPipeConnectionRetryDelay: 250
maxNamedPipeConnectionPoolSize: 512
maxNamedPipePooledConnectionAge: 30000
asyncCompletionThreadCount: 0
initialRequestBufferSize: 4096
maxRequestBufferSize: 65536
watchedFiles: *.js;iisnode.yml
uncFileChangesPollingInterval: 5000
gracefulShutdownTimeout: 60000
loggingEnabled: true
logDirectoryName: iisnode
debuggingEnabled: true
debuggerPortRange: 5058-6058
debuggerPathSegment: debug
maxLogFileSizeInKB: 128
maxTotalLogFileSizeInKB: 1024
maxLogFiles: 20
devErrorsEnabled: true
flushResponse: false
enableXFF: false
promoteServerVars:
Configuration using environment variables
In addition to using web.config and iisnode.yml, you can also configure iisnode by setting environment variables of the IIS worker process. Every setting available in iisnode.yml can also be controlled with environment variables. This option is useful for hosting providers wishing to offer a web based management experience for iisnode.
Integrated management experience
The iisnode module configuration system is integrated with IIS configuration which allows common IIS management tools to be used to manipulate it. In particular, the appcmd.exe management tool that ships with IIS can be used to augment the iismodule configuration. For example, to set the maxProcessCountPerApplication value to 2 for the “Default Web Site/node” application, one can issue the following command:
%systemroot%\system32\inetsrv\appcmd.exe set config "Default Web Site/node" -section:iisnode /maxProcessCountPerApplication:2
This allows for scripting the configuration of node.js applications deployed to IIS.